A Comprehensive Guide to Headless WordPress with Next.js
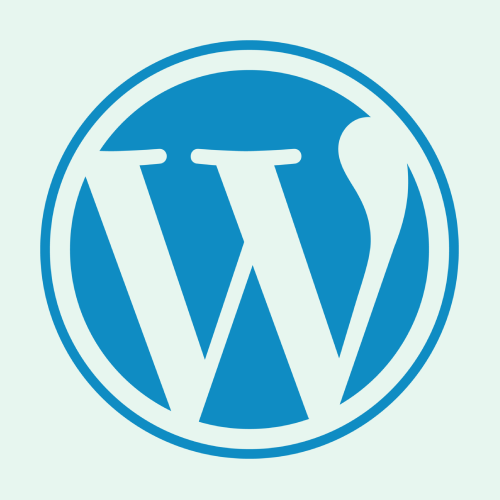
In the evolving world of web development, the separation of the front-end and back-end, known as the “headless” architecture, has gained significant traction. For WordPress developers, integrating this architecture with modern frameworks like Next.js opens up a realm of possibilities, allowing for more dynamic, performant, and scalable websites. In this tutorial, we’ll dive deep into what Headless WordPress is, why it’s advantageous, and how you can get started with integrating WordPress and Next.js.
What is Headless WordPress?
Headless WordPress refers to decoupling the front-end presentation layer from the back-end content management system (CMS). Traditionally, WordPress uses themes and PHP to render the front-end, but in a headless setup, WordPress is used solely as a content repository, while the front-end is built using JavaScript frameworks like Next.js.
Advantages of Headless WordPress
- Performance: By using Next.js, which supports server-side rendering (SSR) and static site generation (SSG), websites load faster and perform better in terms of SEO.
- Flexibility: Developers can use any front-end technology they prefer, such as React, Vue.js, or Angular.
- Scalability: The decoupled architecture allows for easier scaling, as different parts of the website can be managed and scaled independently.
- Security: Since the front-end and back-end are separated, there’s an additional layer of security against attacks targeting the WordPress front-end.
Getting Started: Integrating WordPress with Next.js
Let’s walk through the process of setting up a headless WordPress site using Next.js. This tutorial assumes you have a basic understanding of both WordPress and React.js.
Step 1: Set Up Your WordPress Backend
- Install WordPress: If you don’t have WordPress installed yet, you can set it up on your local machine or on a hosting service.
- Install the REST API Plugin: WordPress comes with a REST API by default, but you may need to install additional plugins like WPGraphQL for a more robust API, especially when working with large datasets.
- Configure Permalinks: Go to
Settings > Permalinks
in the WordPress dashboard and select a permalink structure that suits your needs, as this will affect your API endpoints. - Set Up Custom Post Types and Fields: If your project requires custom content types or fields, you can create them using plugins like Advanced Custom Fields (ACF).
Step 2: Create a Next.js Project
- Install Node.js: Make sure you have Node.js installed on your machine. If not, download and install it from nodejs.org.
- Create a Next.js Application: Open your terminal and run the following commands:
npx create-next-app my-headless-wordpress cd my-headless-wordpress npm run dev
This will create a new Next.js project and start a development server.
- Install Required Dependencies: You’ll need to install the
axios
orgraphql-request
package to fetch data from WordPress. For example:npm install axios
Step 3: Fetch Data from WordPress
- Create an API Fetching Function: Inside your Next.js project, create a file called
lib/api.js
. This will house the function that fetches data from your WordPress site:import axios from 'axios';
const WORDPRESS_API_URL = 'https://your-wordpress-site.com/wp-json/wp/v2'; export async function getPosts() { const response = await axios.get(`${WORDPRESS_API_URL}/posts`); return response.data; } - Fetch Data in Pages: Next.js uses pages to render content. To display WordPress posts, open the
pages/index.js
file and modify it as follows:import { getPosts } from '../lib/api';
export default function Home({ posts }) { return ( <div> <h1>Headless WordPress with Next.js</h1> <ul> {posts.map(post => ( <li key={post.id}>{post.title.rendered}</li> ))} </ul> </div> ); } export async function getStaticProps() { const posts = await getPosts(); return { props: { posts, }, }; }This code fetches the posts during the build time using
getStaticProps
, making your site static and fast.
Step 4: Customize and Optimize
- Styling: Use CSS-in-JS or any preferred styling method to customize the appearance of your site.
- SEO Optimization: Implement SEO best practices by adding meta tags, structured data, and optimizing images. You can also use the
next-seo
package for better SEO handling in Next.js:npm install next-seo
Configure it in your pages:
import { NextSeo } from 'next-seo';
export default function Home({ posts }) { return ( <div> <NextSeo title="Headless WordPress with Next.js" description="A comprehensive guide on how to integrate WordPress with Next.js." /> <h1>Headless WordPress with Next.js</h1> {/* ... */} </div> ); }
Step 5: Deploy Your Site
- Choose a Hosting Provider: You can deploy your site using services like Vercel (the creators of Next.js) or Netlify, both of which provide seamless integration with Next.js.
- Connect to a Git Repository: Push your Next.js project to a Git repository (GitHub, GitLab, etc.) and link it to your hosting provider.
- Deploy: Follow the instructions provided by your hosting service to deploy your application.
Conclusion
Headless WordPress with Next.js is a powerful combination that allows developers to build high-performance, scalable, and modern websites. By separating the front-end from the back-end, you gain more flexibility and control over your site’s functionality and performance.
This tutorial has provided a step-by-step guide to setting up a headless WordPress site using Next.js, from initial setup to deployment. With the information and examples given, you should be well on your way to creating your own headless WordPress projects, opening up new possibilities for what you can achieve with web development.
Feel free to explore further by adding custom features, implementing advanced caching strategies, or integrating additional third-party services to enhance your site. Happy coding!